"One-way" / "Pass-through" Platforms in Phaser 3: Sprites
- cedarcantab
- May 4, 2022
- 3 min read
In this post we explore platforms commonly referred to as "one-way", ie the ones that you can "jump through" from the bottom and land from the top.
We also look at "pass-through" platforms where you can "fall" through when you press the down key from standing position.
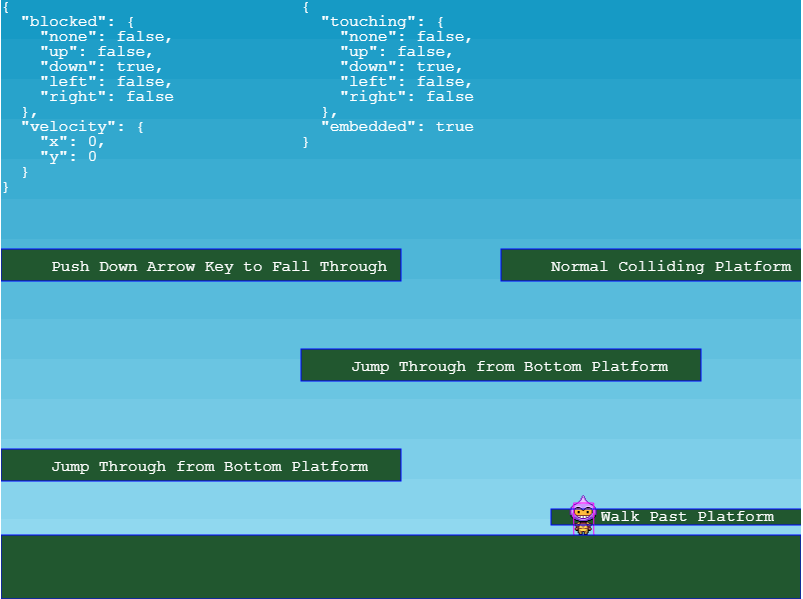
In Phaser 3, the effect is actually very easy to achieve, by utilising the checkCollision property of the physics body given to the platform.
checkCollision :Phaser.Types.Physics.Arcade.ArcadeBodyCollision
According to the documentation here, checkCollision property of the arcade physics body is somewhat concise:
Whether this Body is checked for collisions and for which directions. You can set checkCollision.none = true to disable collision checks.
checkCollison.none = true is simple enough to understand. It simply stops collision checks from taking place, as if there is no physics body.
By clicking on the Phaser.Types.Physics.Arcade.ArcadeBodyCollision type link in the same description, takes you to the following:

With a bit of experimentation, it seems that setting, for example, body.checkCollision.down = false, means that no collision detection is carried out for any other body colliding from the bottom. (It does not mean that the there is no collider a the bottom edge of the rectangle physics body.)
Hence, if you want the player to be able to jump "through" the bottom of a particular platform, through the entire platform, then land on top of the platform, you set the checkCollisions.down property of the particular platform's physics body to false.
this.platforms = this.physics.add.staticGroup();
this.platforms.create(400, 568, 'ground').setScale(2).refreshBody(); // this is the ground
this.platforms.create(500, 250, 'ground').setOrigin(0).refreshBody();
this.add.text(550,260,"Normal Colliding Platform")
this.platforms.create(0,450, 'ground').setOrigin(0).refreshBody()
.body.checkCollision.down = false;
this.add.text(50,460,"Jump Through from Bottom Platform");
this.platforms.create(300, 350, 'ground').setOrigin(0).refreshBody()
.body.checkCollision.down = false;
this.add.text(350,360,"Jump Through from Bottom Platform")
Platforms that you can fall through
So you can have platforms that you can jump through the bottom and land on. But what if you wanted to be able to "fall" through the platform that you are standing on, by pressing the down key? You can do this by "temporarily" setting the "up" property of the checkCollison object to false. Of course, you need a way to reset the property to true, otherwise you will never be able to land on the platform. The simplest way to do this is to reset the property to true when the player jumps - to do this you need to keep track of which platform the player has "disabled" the checkCollision property.
More specifically, you instantiate the platform in the same way as a "normal" platform.
this.passThruPlatforms = this.physics.add.staticGroup();
this.passThruPlatforms.create(0, 250, 'ground').setOrigin(0).refreshBody()
.body.checkCollision.down = false;
this.add.text(50, 260,"Push Down Key to Fall Through")
In my example code, I have created a new group to hold just these type of platforms, so that the "special" collision detection function is called.
this.physics.add.collider(this.player, this.passThruPlatforms, this.onPlatform);
The callback function is defined as follows. All it does is to tell the player object that he is now standing on a "pass through" platform, and which particular platform he is standing on.
onPlatform(player, platform) {
player.isOnPlatform = true;
player.onPlatform = platform;
}
and in the player's controller script, you check for the down arrow key press, and the rest should be self-explanatory.
if ((this.cursors.up.isDown && this.body.touching.down)) {
this.setVelocityY(-400);
if (this.isOnPlatform) {
this.onPlatform.body.checkCollision.up = true;
this.isOnPlatform = false;
this.onPlatform = null;
}
}
if ((this.cursors.down.isDown && this.isOnPlatform)) {
this.onPlatform.body.checkCollision.up = false;
Platforms that you can walk past
Following on from the above, if you set all the "directions" except for up to false, it means that you can walk past the platform from the left or right as well as jump through the bottom of the platform.
The example code is accessible below, for your perusal and comment.
コメント