In part 6, we finally got to the stage of creating something which looks vaguely like a danmaku pattern, albeit very basic. This will form the basis on which to build on in future series.
In this post, we take a breather from building on the danmaku spawner, and add a player ship to the screen that we can move around. This post builds on the danmaku code used in step 6 (we do not amend the danmaku code, except changing the image of the bullet).
Create a new Player object
First, we need a picture for our player ship. As I have no artistic creativity, I have once again poached an image used in many of the examples by Samme of Phaser.Discourse blog, as below. It is a png file which is 60 pixels wide and 50 pixels high.

We use Phaser's load.image method to load the image (no need to change the source - set in game config - from which to load the png file, as it is the same as before).
this.load.image("ship", "assets/sprites/bsquadron1.png");
Then we create the Player class extending the Phaser Arcade Physics Image object. A Phaser image game object is an image with an Arcade Physics body and related Phaser components which we can utilise to make the character move. We can just as easily utilise the sprite object as opposed to an image object, but we have no animation in this example so we'll stick with image object.
class Player extends Phaser.Physics.Arcade.Image {
constructor(scene, x, y, texture) {
super(scene, x, y, texture);
// Add the game object to the scene
scene.add.existing(this);
// Add the physics body to the scene
scene.physics.add.existing(this);
// set body's collider world bounds to true so that the player cannot be moved outside the screen
this.body.collideWorldBounds = true;
// create Phaser object to detect status of cursor keys, Space Bar, and shift
this.cursors = scene.input.keyboard.createCursorKeys();
} // end of constructor
/////// move() method goes here ////////
} // end of Player class
Make the ship movable with the cursor keys
Note that we create a Phaser cursorkey object in the construction function. By adding the following code as the move() method of the Player class, we can move the Player around the screen with the cursor keys, calling the method from the main function update()
move() {
this.setVelocity(0);
// use the cursorkeys object created above to move the ship around
if (this.cursors.left.isDown) {
this.body.velocity.x -= 300;
} else if (this.cursors.right.isDown) {
this.body.velocity.x += 300;
} else if (this.cursors.up.isDown) {
this.body.velocity.y -= 300;
} else if (this.cursors.down.isDown) {
this.body.velocity.y += 300;
}
} // end of move method
Then we can instantiate the Player class from function create()
player = new Player(this, WIDTH / 2, HEIGHT - 100, "ship");
Don't forget to call the move method of the Player class, from the main update function, otherwise the player ship won't move however hard you press the cursor keys!
player.move(); // call the player class move() method so that we can move the player around with the cursor keys
Finally, we change the danmaku bullet image to "bullet8" when we instantiate the Danmakuwrapper, including below in the config.
bulletType: "bullet8" // specify the bullet image to be used
And that's it! We now have player ship that you can move around the screen with the cursor keys.
The entire code is available as CodePen Pen for your perusal.
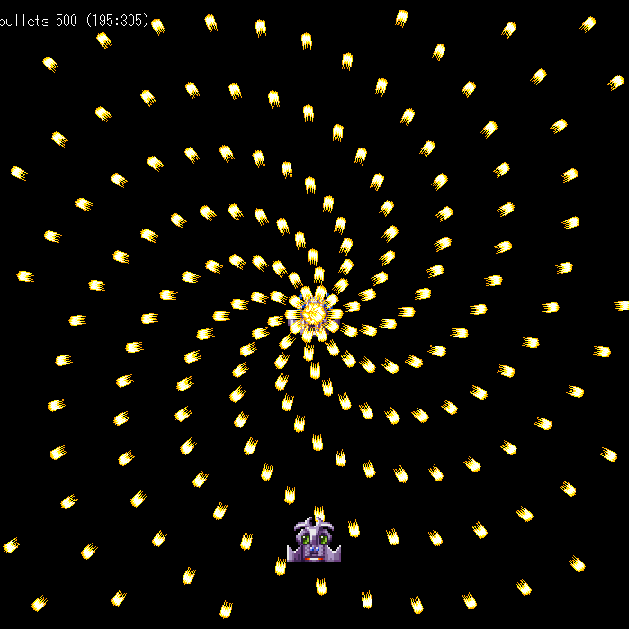
Comments