Danmaku using Phaser 3 (step 6): Multiple Spiral
- cedarcantab
- Sep 2, 2021
- 2 min read
Updated: Oct 8, 2021
In examples so far the Danmaku fired one stream of bullets. The stream might have rotated or the bullets might have been fired on intermittent basis, but in essence, only one stream of bullets. Think of it like having one cannon from which to fire. The cannon may rapid fire a stream of bullets, but there is only one cannon. But what if the Danmaku had multiple cannons to fire from? Such an addition should be relatively straight forward.
First we enable the number of cannons to be passed as a parameter to the Danmaku class, when it is instantiated, like so (for this example, we've chosen to have 10 cannons). The parameters have been reordered from previous examples (doesn't make any difference to fundamentals - just made it easier for myself to read) and the bullet image has been changed again (but from the 10 bullet images always loaded in the script - so no need to change the this.load.image code in the preload function).
danmaku = new DanmakuWrapper(this, {
x: enemy.x, // origin of the danmaku spawn
y: enemy.y, // origin of the danmaku spawn
shootAngle: 90, // direction in which the cannon faces, ie bullet direction.
angleVelocity: 90, // angular velocity of the 'cannon' that fires the bullets
numberOfCannons: 10, // number of cannons
bulletSpeed: 200, // speed of bullets fired from cannon
bulletAcceleration: 0, // acceleration of bullets fired from cannon
timeBetweenBullets: 100, // time between bullets in ms
bulletType: "bullet3" // specify the bullet image to be used
});
After that, add one line in the DanmakuWrapper class constructor function to read the number of cannons parameter.
this.numberOfCannons = config.numberOfCannons || 1; // number of cannons. default is 1.
Then all we need to do is to execute the bullet firing section of the fireweapon method 'number of cannons' number of times, using a for loop. The for loop simply starts from this.angle (which is set by bulletAngle parameter passed from the danmaku class) and rotates a full circle in increments of 360 divided by the number of cannons.
for (let i = 0; i < this.numberOfCannons; i++) {
const bullet = bullets.getFirstDead(false);
if (bullet) {
const direction = this.angle + i * (360 / this.numberOfCannons);
bullet.fire({
x: this.danmakuPosX, // the bullet is fired from the middle of the Danmaku class (ie the spawner)
y: this.danmakuPosY, //
shootAngle: direction, // direction in which the bullet is fired
bulletSpeed: this.bulletSpeed, // the speed of the bullet
bulletAcceleration: this.bulletAcceleration,
bulletAngle: direction, // make the bullet face the direction in which it is fired
bulletAngleVelocity: this.bulletAngleVelocity,
bulletType: this.bulletType
}); // end of bullet fire method
} // end of if statement which checks whether bullet is null
} // end of for loop
And that's it!
The entire code is available as the following Codepen Pen, for your perusal.
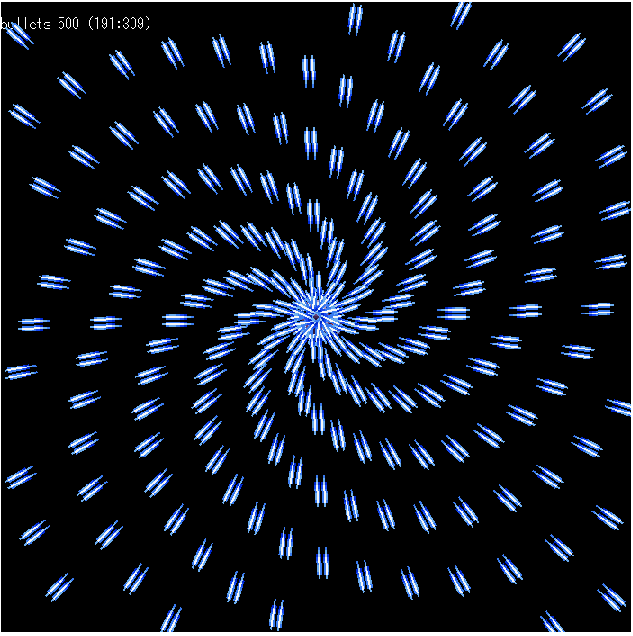
Comentarios