Danmaku using Phaser 3 (step 3): "Bending" Bullets
- cedarcantab
- Aug 29, 2021
- 2 min read
Updated: Oct 8, 2021
In this example we briefly step away from the bullet firing pattern and fiddle with the bullet class itself.
I mentioned in step 1 that the bullet itself is a game object with an arcade physics Body. I have utilised various members of the game object/Body to achieve several effects, simplest one being to make it fly across the screen by setting the Velocity of the object. I have also set the "onWorldBounds" of the Body to true to make it disappear 'automatically' when it flies outside the screen.
However, there are many other members that we can utilise to achieve other effects. For example, just as we used the 'angle' and 'angularVelocity' of the Danmaku object/Body to adjust the direction in which bullets are fired, we can utilise the same members of the bullet itself to, for example, make the bullet 'face' the direction in it is flying. Taking the example from step 2, I simply add the following 2 lines to the Bullet class constructor function. I have set up the Bullet class with the parameter set up as a Javascript object so that it is very easy to make these type of changes.
this.angle = config.bulletAngle || 0;
this.body.angularVelocity = config.bulletAngleVelocity || 0;
Then I simply need to add these new parameters when I call the Bullet.fire() method from the fireweapon() method of the DanmakuWrapper class. The only changes are the 2 lines in bold.
fireweapon(elapsedTime) {
const bullet = bullets.getFirstDead(false);
if (bullet) {
bullet.fire({
x: this.danmakuPosX,
y: this.danmakuPosY,
shootAngle: this.angle,
bulletSpeed: this.bulletSpeed,
bulletAngle: this.angle,
bulletAngleVelocity: this.bulletAngleVelocity
});
} // end of fire method
} // end of fireweapon method
The angular velocity of the bullet should be set when the DanmakuWrapper class is instantiated, as follows. In the example, the angular velocity is set to 360 degrees, ie bullet rotates 360 degrees every second.
danmaku = new DanmakuWrapper(this, {
x: enemy.x, // origin of the danmaku spawn
y: enemy.y, // origin of the danmaku spawn
shootAngle: 90, // set shoot angle to 90, which is downwards
bulletSpeed: 200, // bullet speed
timeBetweenBullets: 100, // time between bullets in ms
angleVelocity: 45, // angular velocity of the 'cannon' that fires the bullets
bulletAngleVelocity: 360 // speed at which individual bullets rotate themselves
});
Finally, we change the image of the bullet. In the previous examples, I was using a circle as the bullet image. We change that to an elongated image ("bullet6" - remember, we had built the code to load in 10 different bullet images?) by changing the key when we create the bullets group.
bullets.createMultiple({
classType: Bullet,
frameQuantity: 500,
active: false,
visible: false,
key: "bullet6"
});
Then voila! Bullets rotating as they fly! In the example, angular velocity is set to 360 which means that the bullet rotates 360 degrees clockwise every 1 second. If you set the bulletAngleVelocity to 0, then the bullets will 'face' the direction in which they fly and not rotate.
The entire code is available for your perusal in the below Pen.
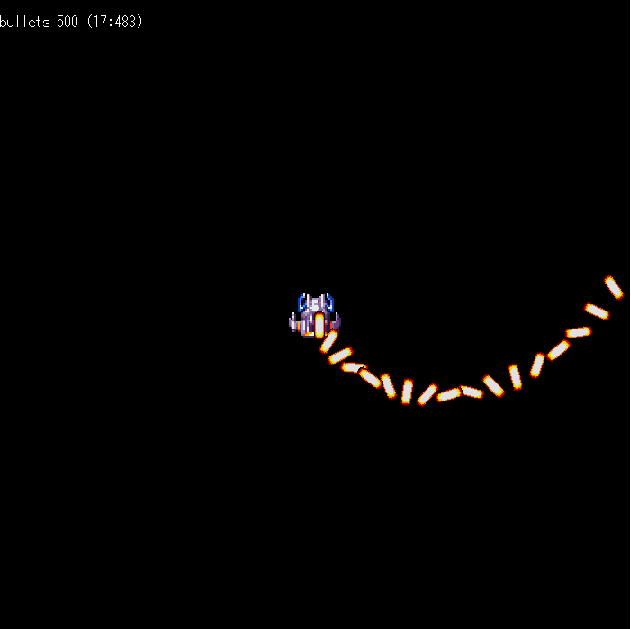
Commentaires