Danmaku using Phaser 3 (step 20): Waving Bullets!
- cedarcantab
- Oct 9, 2021
- 3 min read
Updated: Oct 14, 2021
I have spent a lot of my time so far on manipulating the angles of the cannons of the danmaku object, thereby spraying the bullets in different directions. More recently, I have also started manipulating the speed of the bullets, like in SPREAD shots, or overtaking bullets, again by adding more functionality to the danamku object itself.
There is of course, another very important object; and that is the individual bullets themselves. At the simplest level, once they are fired by the danmaku, they just fly in a straight line. There is already a fair bit of functionality to the bullet class itself. Among other things, it can accelerate (at the accelerate rate pre-defined by the danmaku) and it can fly along a curve (the degree of which is predetermined by the danmaku). In a more recent addition we have created a bullet that actively "seeks" a target.
In this post, we add another functionality to the bullet itself - the bullet will fly along a sine wave (actually, it is not strictly a sine curve but something that looks like a sine wave).
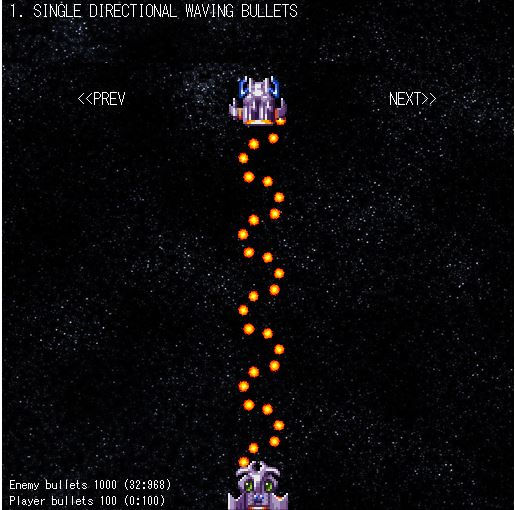
In principle, what we want to do is to adjust the direction of the bullet by incremental amounts as it flies along, and reset the velocity vector accordingly as the bullet flies along. In the "BEND" bullettype, the direction of the bullet was controlled by the angularVelocity of the bullet object; in that case it was relatively straightforward since all you needed to do was set the angularVelocity to some value and the angle of the physics body would be adjusted automatically, and the velocity of the physics body could be re-calculated based on the rotation of the physics body.
In the case of a "waving" bullet, we can again utilise the physics body's rotation, but it cannot be moved in a single direction. Instead, it moves from -90 degrees to 90, and then back again. We can use tweens to "count" do this. The below code will create a "counter" that will count from -90 to 90, and then backwards, then repeat. We have also introduced a new variable called bulletWaveCycle which determines how quickly (or slowly) the heading the bullet changes from left to right.
if (this.bulletType === "WAVING") {
this.swing = this.scene.tweens.addCounter({
from: -90,
to: 90,
duration: this.bulletWaveCycle,
repeat: -1,
yoyo: true
})
}
We can add this "counter" to the initial direction of the bullet, which is stored in this.shootAngle, so that the bullet flies in the intended direction, but "waving" as it flies. In the sense that the velocity vector is adjusted based on the rotation of the physics body as the bullet flies, there are similiarities to both the "BEND" type and the "HOMING" type of bullet. We will use the "bulletType" parameter to tell the bullet object how it should fly.
In the preUpdate function, we will include the following:
switch (this.bulletType) {
case "BEND":
this.adjustVelocity()
break;
case "HOMING":
this.seek();
this.adjustVelocity();
break;
case "WAVING":
this.angle = this.shootAngle + this.swing.getValue();
this.adjustVelocity();
break;
default:
break;
} // end of bulletType switch statement
The important bit is the highlighted line. This fetches the "counter" value using swing.getValue, and adds this value to the shootAngle, and resets the rotation of the physics body to the result.
We have created a new function called adjustVelocity to reset the velocity vector of the bullet based on the current angle of the bullet physics body.
The danmaku pictured at the beginning of this post was created with the following parameters:
danmakuPattern.push({
name: "SINGLE DIRECTIONAL WAVING BULLETS",
danmakuType: "NWAY",
numberOfCannons: 1,
cannonAngle: 90,
numberOfShots: -1,
bulletType: "WAVING",
bulletWaveCycle: 300,
bulletSpeed: 200, // speed of bullets fired from cannon
timeBetweenShots: 100,
bulletTexture: "bullet7"
});
But you can have NWAY or Mutliple-NWAY shoot waving bullets, like the 4 x 2-way danmaku below. You can have the below danmaku rotate as well to create a multi-spiral danmaku with waving bullets.
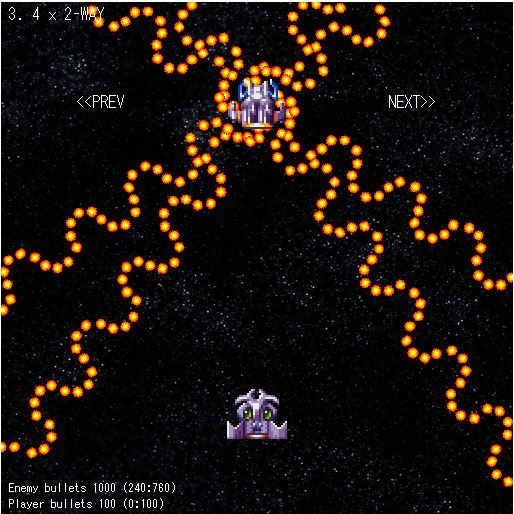
Limitations of the existing code
What I cannot do with this version of the code is to have bending bullets that "wave", as I have used one variable to indicate whether a bullet is BEND, WAVING or HOMING.
As a reminder to myself, to create a bullet that flies along a curve, but waves as it does so would require a fair bit of re-write, requiring another variable to track the "overall" direction of the bullet's heading, and this would need to be adjusted by the counter. That in itself would not be too difficult, but when I get round to do that, I will probably re-write the BEND bullet code, so that "curve" of the bullet flight path would be independent of the physics body of the object - that would allow, for example to have bullets that (the image) rotate and fly along a curve.
As always, here is the CodePen, for your perusal.
コメント