Danmaku using Phaser 3 (lesson 35): Angle Range Step revisited
- cedarcantab
- Nov 5, 2021
- 3 min read
Updated: Nov 23, 2021
This will be a short post as it will not add any fundamentally new danmaku. Rather, it is more a reminder to myself of what I have done. It is making the angle step functionality a little bit easier to handle.
In the previous version of the code which has remained unchanged for a long time, the parameter is an object of its own. It was one of the first times that I started using objects as parameters. Since then I have gradually "grouped" parameters into 2 main ones: danmakuConfig and bulletType. cannonAngleRange remains as an object type parameter of its own. It is also somewhat cumbersome in that even if the parameter is a static number (as opposed to one that steps through a range of ranges) it requires it to be set like cannonAngleRange: {start: XX}, as opposed to simply cannonAngleRange: XX.
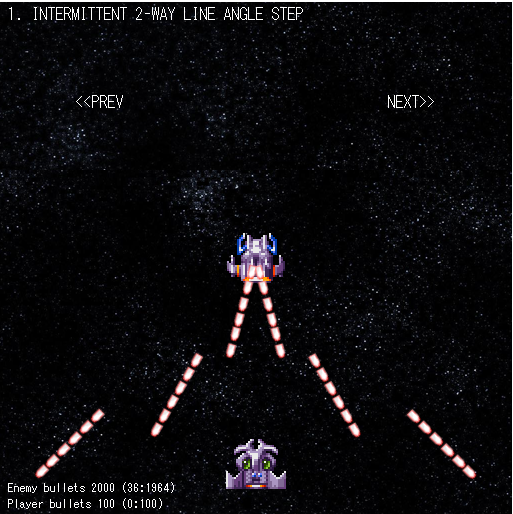
Changing the parameter names again
As stated above, in the previous version of the code, this was a "separate" parameter set as a object in terms of angleRange: {start: XX, step: XX, end: XX}. To make it a little bit easier to read and remember, I have:
set this as a property of the cannonConfig object parameter called cannonConfig.angleRange, and
if the property is given a number, then that number will automatically be assigned to this.cannonAngleRangeStart, otherwise if it is given an object in the form of {start: XX, step: XX, end: XX}, then the code will work very similar to the original code.
Probably easier to figure out what I have done by looking at the danmaku.setProperties function that "reads" the parameter.
// cannonConfig angleRange parameter
switch (typeof(cannonConfig.angleRange)) {
case "number":
this.cannonAngleRangeStart = Phaser.Math.DegToRad(cannonConfig.angleRange);
break;
case "object":
this.cannonAngleRangeStart = Phaser.Math.DegToRad(cannonConfig.angleRange.start) || 2 * Math.PI;
this.cannonAngleRangeStep = Phaser.Math.DegToRad(cannonConfig.angleRange.step) || 0;
this.cannonAngleRangeEnd = Phaser.Math.DegToRad(cannonConfig.angleRange.end);
break;
default:
this.cannonAngleRangeStart = Math.PI*2;
this.cannonAngleRangeStep = 0;
}
this.cannonAngleRangeRef = this.cannonAngleRangeStart;
if (!this.cannonAngleRangeEnd) {
this.cannonAngleRangeEnd = this.cannonAngleRangeStep > 0 ? 2 * Math.PI : 0;
}
The new code first checks the datatype of the parameter and assigns the values to the various - from here the code remains much the same.
Main logic of stepping through the angle ranges
The following code in the fireShot function checks whether we need to step through a set of angle ranges, as opposed to remain static. I have created a new variable called this.repeatShotsCount which is set to the this.repeatShotsTimer.repeatCount at the beginning of fireShot function each time it is called.
if (
this.cannonAngleRangeStep !== 0 &&
this.repeatShotsCount === this.numberOfShots
) {
this.cannonStepThruAngleRange();
}
I have also added a yoyo option to the anglerange step. How I achieved it, is easier to understand by looking at the main cannonStepThruAngleRange function.
cannonStepThruAngleRange() {
this.cannonAngleRangeRef += this.cannonAngleRangeStep;
switch (Math.sign(this.cannonAngleRangeStep)) {
case 1:
if (this.cannonAngleRangeRef >= Math.min(Math.PI * 2, this.cannonAngleRangeEnd)) {
if (!this.cannonAngleRangeYoYo) {
this.cannonAngleRangeRef = this.cannonAngleRangeStart;
} else {
this.cannonAngleRangeRef = Math.min(Math.PI * 2, this.cannonAngleRangeEnd);
this.cannonAngleRangeStep *= -1;
[this.cannonAngleRangeStart,this.cannonAngleRangeEnd] = [this.cannonAngleRangeEnd,this.cannonAngleRangeStart]
}
}
break;
default:
if (this.cannonAngleRangeRef <= Math.max(0, this.cannonAngleRangeEnd)) {
if (!this.cannonAngleRangeYoYo) {
this.cannonAngleRangeRef = this.cannonAngleRangeStart;
} else {
this.cannonAngleRangeRef = Math.max(0, this.cannonAngleRangeEnd);
this.cannonAngleRangeStep *= -1;
[this.cannonAngleRangeStart,this.cannonAngleRangeEnd] = [this.cannonAngleRangeEnd,this.cannonAngleRangeStart]
}
}
}
} // step through angle ranges
I was quite pleased with myself for being able to swap the value of two variables (this.cannonRangeStart and this.cannonAngleRangeEnd) in 1 line. However, having written the code, I now realise it would have been much easier to use tweens, particularly as I have found out that there is a "stepped" ease option in Phaser 3 tweens (see example here) - this is something for a future date.
Comments