In the previous post I created functions to draw heart, spade, club and diamond, utilising Phaser's Curve.Path object. As explained before, the Curve.Path object is a series of straight lines and curves, and there are a variety of ways of defining the curve like ellipses, quadratic Bezier curve and cubic Bezier curve. That in itself is very powerful in itself, but what is interesting about the Curve.Path object is that the "elements" making up the path do not have to be joined up, i.e. whatever picture we create does not have to consist of one continuous line. In this post we utilise this feature to draw Smiley faces.
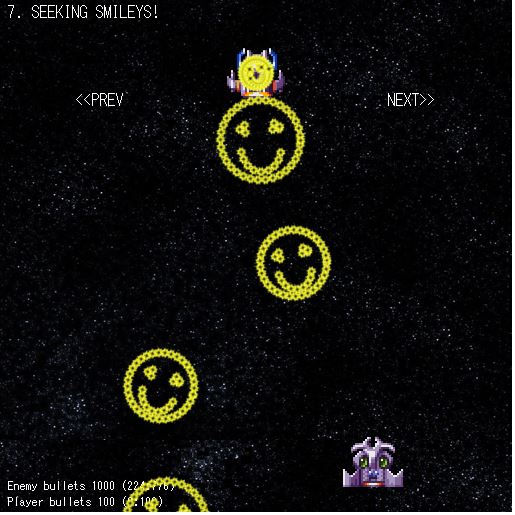
There is no new technique used here, except the judicious use of moveTo which essentially lifts up the "pen" and moves its to another spot on the canvas.
function createSmiley(heading, offset) {
const angle = heading - Math.PI/2;
const nominalSize = 100;
const radius = nominalSize / 2;
const centre = new Phaser.Math.Vector2(offset.x, offset.y);
const start = new Phaser.Math.Vector2(radius,0).rotate(angle).add(centre)
const smiley = new Phaser.Curves.Path().moveTo(start);
// draw outline of face
smiley.ellipseTo(radius,radius,0,360,false,Phaser.Math.RadToDeg(angle));
// draw mouth
smiley.moveTo(new Phaser.Math.Vector2(radius*0.5,radius*0.2).rotate(angle).add(centre));
smiley.ellipseTo(radius*0.5, radius*0.6, 0,180,false, Phaser.Math.RadToDeg(angle));
// draw left eye
smiley.moveTo(new Phaser.Math.Vector2(-radius*0.3, -radius*0.3).rotate(angle).add(centre));
smiley.ellipseTo(radius*0.1,radius*0.1,0,360,false,Phaser.Math.RadToDeg(angle));
// draw right eye
smiley.moveTo(new Phaser.Math.Vector2(radius*0.5, -radius*0.3).rotate(angle).add(centre));
smiley.ellipseTo(radius*0.1,radius*0.1,0,360,false,Phaser.Math.RadToDeg(angle));
return smiley
} // end of function to create Smiley face
Given the nature of the picture, the code is actually simpler than those of say spade or club; requiring no use of Bezier curves. The code to call the function is as below - in principle the same as the code for drawing the star, 2 posts ago.
case "SMILEY":
const smiley = createSmiley(this.rotation, this.danmakuPosition);
cannonsFromCurve(
smiley, // this is the polygon object on which danmaku pattern will be based
this.danmakuPosition, // this is the "centre" of polygon from which the cannonshot speeds will be calibrated
this.danmakuCount, // number of bullets to create polygon
this.bulletSpeed,
this.cannonAngles, // this is the array to hold the cannon angles - pass by reference
this.cannonShotSpeeds // this is the array to hold the cannon shoot speeds - pass by reference
);
break;
Combing with stop & go bullets
The "standard" way of drawing a smiley that expands outwards from a point, is with the parameter set below.
danmakuPattern.push({
name: "SMILEY",
danmakuConfig: { class: "DRAW", type: "SMILEY",count:100,angle:90 },
numberOfShots: -1,
bulletType: {
class: "NORMAL" ,
speed: 200, fireRate: 2500, texture: "roundSML", frame: 3
},
});

You can combined the DRAW danmaku with danmakuTarget set to the player and stop & go bullets to create smiley faces that "fly" towards the player. The danmaku pictured at the top of this post was created with the parameter set below.
danmakuPattern.push({
name: "SEEKING SMILEYS!",
danmakuConfig: { class: "DRAW", type: "SMILEY",count:50,angle:90 },
numberOfShots: -1,
cannonTarget: player,
bulletType: {
class: "NORMAL" ,
speed: 800, fireRate: 650, texture: "cross", frame: 3
},
bulletTransform: {
type: "STOP&GO",
stage1Time: 80,
stage2Time: 250,
bearing: 90,
speed: 200,
bearingLock: true,
texture: "cross",
frame: 3,
lifeSpan: 10000,
},
});
And that's that! DRAW danmaku code is complete..until the next time!
Here's the CodePen for your perusal and comment.
Comments