Danmaku using Phaser 3 (lesson 29): ZigZag bullets
- cedarcantab
- Oct 20, 2021
- 3 min read
In this post, we give the bullet another ability - the ability to zig and zag.
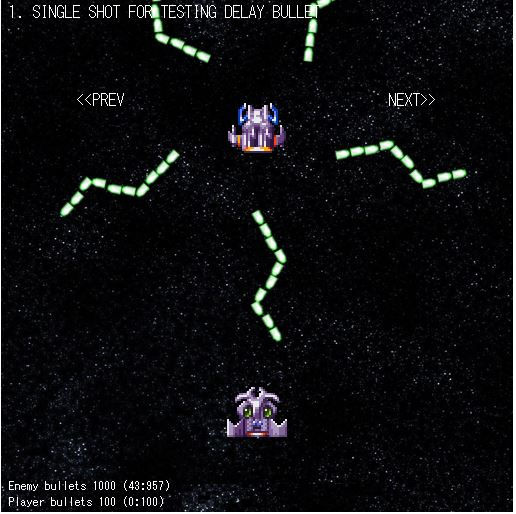
But before we get to the zigzag code...
This is becoming a bit of a habit, but I have once again made changes to how the parameters are passed to the danmaku, to hopefully make the parameters easier to handle. Firstly, I have changed the name of some of the parameters, the key change being to change danmakuType to danmakuConfig.
I have then taken many of the primitive parameters to properties of the above, as follows:
cannonAngle: danmakuConfig.angle (within the danmaku object, changed name of this.cannonAngle to this.danmakuAngle)
cannonAngleLock: danmakuConfig.angleLock
As an aside, for MULTI_NWAY, I have changed the property to determined the number of NWAYs from count to size, and now use danmakuConfig.count to replace the numberOfCannons variable .
I have also changed the name of danmakuType "POLYGON" to "DRAW", as I intend to add functionality to this danmakuType in the future to draw more than just regular polygons. Previously I used danmakuConfig.count as the number of vertices, but have now changed danmakuConfig.size for POLYGON (which is now DRAW).
Refactoring of the adjustVelocity of bullet method
As we had very similar code to reset the velocity vector of the bullet in the setMotion method (called at the beginning of bullet.fire) and adjustVelocity (called by various bullet types, such as bending bullets), I refactored it and in so doing, increased the parameter set accepted by the adjustVelocity function. I also added a line to the adjustVelocity function to rotate the image of the bullet to the new direction, unless the bullet is a rotating type.
adjustVelocity(targetAngle, speed, acceleration) {
this.scene.physics.velocityFromRotation(
targetAngle,
speed,
this.body.velocity
); // reset velocity as bullet curves
this.scene.physics.velocityFromRotation(
targetAngle,
acceleration,
this.body.acceleration
);
if (this.bulletAngularVelocity === 0) {
this.setRotation(targetAngle);
}
}
There are more changes I want to make (eg make shotType into an object) but I am not going to push my luck too far, as I have made a lot of fundamental changes in one go.
Now onto creating ZigZag bullets
As this requires changing the behaviuor of the bullet at fixed intervals after firing, we will use the bulletTransform parameter to configure how the bullet should zigzag. We need to tell it how much to change the bearing angle; for this we will create a property called bearingDelta. Otherwise, we will use the stage1Time property to tell the bullet how quickly to zig and zag.
I also create a new variable called this.bulletToggle, which essentially flips between +1 and -1, to use to toggle the direction of the bullet.
We want a timer to measure when to change direction; for this we will use timeSince Fired. However, I have also changed the name of timeSinceFired to bulletTimer, since for the zigzag bullet we will reset this variable to zero every time it changes direction.
The code to make the bullet zig and zag is to be included in the switch statement which gets excecuted when the bulletTimer reaches the stage1Time and is relatively straightforward, as below.
case "ZIGZAG":
this.transformed = false; // for ZIGZAG want the transformed to remain false since transform must continue to be checked
this.bulletTimer = 0; // but reset timer, so that start counting again for next direction change
this.bulletToggle = this.bulletToggle * -1;
const newDirection = this.bulletBearing + this.newBulletBearingDelta * this.bulletToggle;
this.setRotation(newDirection);
this.adjustVelocity(newDirection, this.bulletSpeed, this.bulletAcceleration);
break;
The parameter set to create the danmku at the top of this post is as shown below.
danmakuPattern.push({
name: "5-WAY WITH ZIGZAG BULLETS",
danmakuConfig: {
class: "NWAY" ,count:5, angle: 90
},
cannonAngleRange: {start: 360},
numberOfShots: 10, // how many times to shoot the weapon in succession
stopShotsTime: 3000,
bulletType: {
class: "NORMAL",
speed: 100, fireRate: 150,
target: player,
texture: "shell", frame: 1,
},
bulletTransform: {
type: "ZIGZAG", bearingDelta: 30,
stage1Time: 500,
},
});
As always, here's the CodePen for your perusal and comment.
Comments