In lesson 10, I created a "n-Way" danmaku, where you could specify not only the 'n' (ie the number of cannons spewing out bullets), but also the range of directions in which the n cannons would face. If the range of cannon directions were set to 360 degrees, one would end up with a "Circle" danmaku.
I also added the ability to rotate the entire set of cannons at a predetermined speed, thereby creating a "Rolling n-Way" danmaku. And in lesson 11, I built on the same code by adding the option of adding an element of randomness to the bullet speed and direction, allowing us to generate "Random n-Way" danmaku and (if the cannon angle range is set to 360 degrees) "Random Circle" danmaku.
In this lesson I will try build on the lesson 11 code to give the danmaku object the ability to "wave".
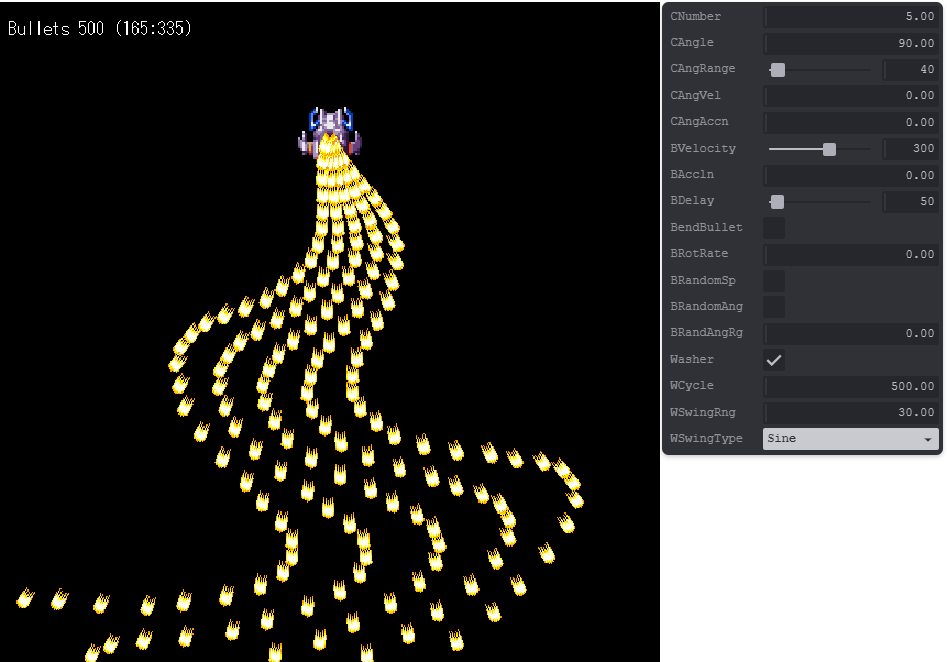
To achieve the desired effect, we can make use of Phaser's tweens, like we did in lesson 8 when I created the "Washer Spiral". A tween is a Phaser object which allows you to manipulate Phaser game objects properties. In essence what I want to do is to change the angle of the danmaku object (ie the cannon angle) across a pre-determined range, in a pretermined timeframe. This is very easy for the Phaser tween. In addition, the Phaser tween gives you the ability to "ease" in lots of different manners.
As in keeping with my more recent trend, rather than create an entirely new Danmaku object, we will "add" the new feature to the existing object and give it the ability to "switch" to a waving (or washer type) to a non-waving type using a flag.
I won't bother going into the details of creating the flag, since we have done similar code in previous examples. Instead the key code to "swing" the bullet streams is the following Tween.
setWasher() { // this function creates the Tween object
this.angle = this.cannonAngle; // reset the angle of bullet to the cannonAngle
this.body.angularVelocity = 0; // if swing type, cannon angle velocity must be zero
this.washerTween = this.scene.tweens.add({
targets: this,
angle: {
from: this.cannonAngle - this.washer.swingRange /2,
to: this.cannonAngle + this.washer.swingRange /2
},
duration: this.washer.cycleLength,
ease: this.washer.swingType,
repeat: -1,
yoyo: true
}); // end of tween which adjusts the angle
} // end of setWasher function
This function is called from the constructor function, if the property to assess whether this is a waving type of danmaku or not is set to true.
// create a tween to swing the cannon (centered around the primary cannon), if washer type is selected
if (this.washer.status) {
this.setWasher();
} // end of if statement to check whether washer type is true
And that's pretty much all of the important bits. Easy, with the incredible power of the Phaser 3 framework.
In fact, I spent a lot more time developing the tweak pane, which now has a lot more variables that you can manipulate in real time, making danmaku experimentation much more enjoyable!
Even with the relatively small number of parameters, there are a dramatic variety of patterns you can generate.
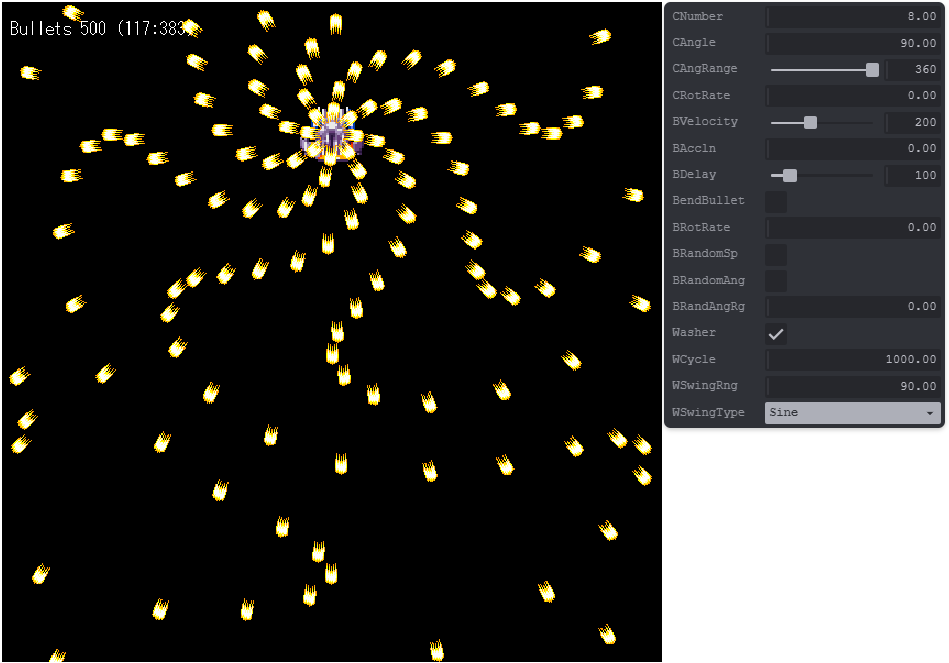
As usual, here is the entire code in CodePen Pen, for your persual and critique.
Comments