What if we gave the bullets a bit of "randomness"? In this post I look at how I added randomness to the fire interval of bullets and their direction to the Rolling n-Way danmaku I created in the previous post.
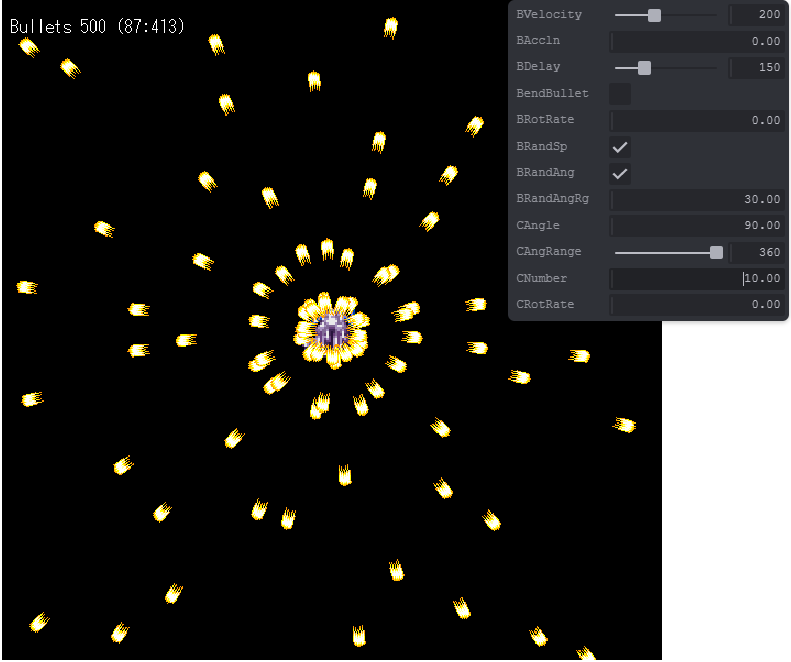
Adding randomness to the bullet fire interval
The consecutive firing of bullets is controlled by a Phaser timer event, created under the name of this.machineGun within the constructor function of the DanmakuWrapper. The delay between each bullet is set by 'delay: this.timeBetweenBullets'. All we need to do is change this delay property of the timer event, every time we fire the bullet. We can do this in the fireweapon function of the danmaku object. We will also create a new property for the danmaku object called 'randomBulletDelay' which will act as a boolean flag to tell whether we should randomise or not. We simply add the following line at the beginning of the fireweapon method.
if (this.randomBulletDelay) {
this.machineGun.delay = this.timeBetweenBullets + Phaser.Math.Between(-this.timeBetweenBullets,this.timeBetweenBullets);
} // to randomise the fire interval of bullets or not
This will add a random number between minus this.timeBetweenBullets and positive this.timeBetweenBullets to the prescribed fire bullet interval, and set the delay timer to the new time.
Adding randomness to the bullet direction
We will create another new property for the danmaku object called 'randomBulletAngle' which will tell if and how the bullet direction should be randomised.
We will use the following function to calculate the "randomness" in bullet direction
randomiseDirection() { // function to return a "random element" to add to the bullet angle
if (this.randomBulletAngle.status) {
return Phaser.Math.Between(-this.randomBulletAngle.range /2, this.randomBulletAngle.range /2)
}
else
return 0;
} // end of randomiseDirection function
Then all we have to do is add what's returned from the above function to bits of our original code that calculates the bullet angle. The bold highlighted bits are the additions.
// calculate the direction of each cannon and store in an array
const cannonAngles = [];
if (this.numberOfCannons <= 1) { // single cannon
cannonAngles[0] = this.angle + this.randomiseDirection();
} // end of calculating angle for where there is only 1 cannon
else {
if (this.cannonAngleRange < 360) { // there is more than 1 cannon and angle < 360
for (let i = 0; i < this.numberOfCannons; i++) {
cannonAngles[i] = this.angle + (i / (this.numberOfCannons-1) - 0.5) * this.cannonAngleRange + this.randomiseDirection();
} // end of for loop where cannon range is less than 360
} // end of if statement checking cannons > 1 && cannonAngle Range < 360
else { // get here if there's more than 1 cannon, and Cannon angle range is 360
for (let i = 0; i < this.numberOfCannons; i++) {
cannonAngles[i] = this.angle + (360 / this.numberOfCannons) * i + this.randomiseDirection();
} // end of for loop where cannon range = 360
}
}
And that's it! The entire code is available in the following CodePen for your perusal.
Comments