in lesson 10 I created a danmaku type that "aimed" its cannons at the player. The code was written such that the direction of the cannon would be adjusted each time the fireShot function was called. So if the player was moving, the stream of bullets would "bend" as the direction of each shot would be adjusted to follow the player. For the same reason, in any rotating type of danmaku, the bullet streams would "bend" with each successive shot being fired at incrementally different angles. But what if we wanted rotating "straight" lines of bullets to be fired? That is the subject of this post.
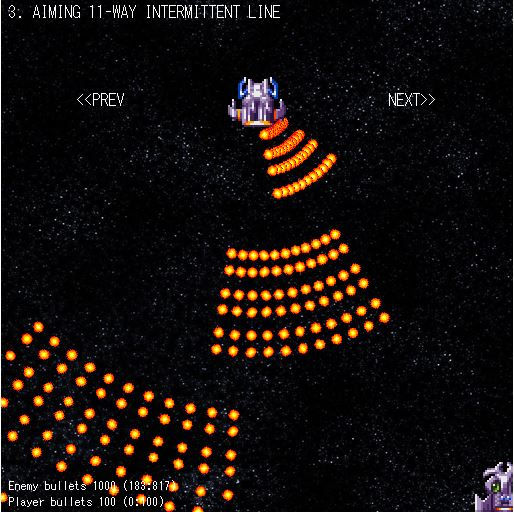
Current version of code adjust the cannon angle every shot
As mentioned above, the cannon angle is adjusted each time the fireShot function is called. For this reason, with the aim parameter switched on, the danmaku looks like below, if the player is moving.
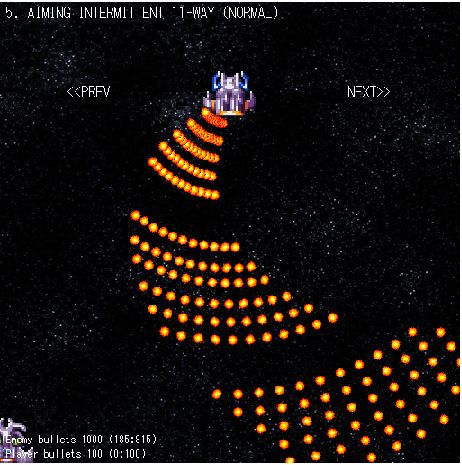
Another important aspect of the current code is that the direction of the cannon is inherently tied to the physics body of the danmaku object, and we have utilised the physics engine to manipulate the angle, such as angularVelocity to say "automatically" rotate the cannon.
However, we now want the option to change the direction of the cannon, only when we want to change it. For example, if we could only "reset" the cannon angle at the completion of each repeatShotCycle (in a intermittent type of danmaku) as opposed to continuously, then we would be able to create straight lines of bullets in each repeatShots cycle. To do this, we need to "separate" the cannon angle from the angle of the physics body; for this we create a new variable called this.referenceAngle and we use this to set the angle of the cannon, as opposed to referring to the physics body angle directly.
In order to tell the danmaku whether to adjust this referenceAngle every shot, or only once each repeatShot cycle, we create another variable called cannonAngleLock. The default for this shall be set to false, ie cannon angle will be reset every shot. If true, we only adjust the referenceAngle at the beginning of each repeatShotsTimer loop to the physics body angle. The result is that if the danmaku physics body is rotating, the bullets would fire in the same direction during one repeatShot cycle, and then reset to the new physics body angle for the next repeatShot cycle.
Implication for the Aim function
In the existing version of the code, there is a parameter called target. The fireShot function checks this variable each time it is called to see if it has been assigned a game object (i.e. the player sprite). If it is, then the physics body angle of the danmaku object is adjusted in the direction of the game object by calling the aimAt function.
aimAt(target) {
// this function aligns the primary cannon in the direction of the target
const targetAngle = Phaser.Math.Angle.Between(
this.x,
this.y,
target.x,
target.y
);
this.setRotation(targetAngle);
} // end of aimAt function
} // end of Core Danmaku class
However, with the above change, the direction of the cannon is determined to this.referenAngle which, if the anglelock variable is set to true, is only set to the physics body angle only once each repeatShot cycle; ie the bullets in each repeatShot cycle will fire in a straight line.
And that's it.
Here is the CodePen for your perusal and comment.
Comments