In the previous post, we gave the player the ability to shoot at the Big Boss alien space ship which shoots rockets in a multiple spiral pattern.
The background is completely black. Devoid of any stars. Would it be nice if we had some stars? That would be easy to do just by adding a picture of space to the scene. As an added touch, let's try to have the stars 'move' like a moving field of stars, ie create a scrolling starfield background.
Several different ways of scrolling a bunch of background stars
The most intuitively obvious method is to simply create a lot of stars as sprite or image game objects, and make them move, just like we have done with the danmaku bullets.
Using TileSprite
Another way is to use something called a tilesprite. According to Phaser documentation "A TileSprite is a sprite that has a repeating texture. The texture can be scrolled... and... automatically wrap and are designed so that you can create game backdrops using seamless textures as a source". Sounds perfect.
First, we need a "seamless texture" to use as a source. Once again, we will "poach" a picture from the Phaser.labs asset folder, and load the relevant starfield image with the following code inserted into the preload function.
// load image of space with lots of stars
this.load.image("starfield", "assets/skies/starfield.png"); // image size is 512x512
We then use the following code within the create function to "draw" the background image. The following code should come at the beginning of the create function, before the other objects are drawn on the screen. Otherwise, the star background will overwrite anything that is drawn before it.
stars = this.add.tileSprite(WIDTH/2,HEIGHT/2,512,512,"starfield")
According to the Phaser docs, the config parameters are:
var image = scene.add.tileSprite(x, y, width, height, textureKey);
"width" and "height" are simply the width and height of the tileSprite in pixels. In our example, the sprite is 512x512. The confusing part are the first 2 parameters. This is with respect to the centre of the tilesprite, ie which are the x and y coordinates where the tilesprite is supposed to be positioned. Hence, the above code is drawing the tilesprite such that the middle of the tilesprite is positioned at WIDTH/2 and HEIGHT/2.
Oh, to make this work effectively, the tilesprite must be the same size as the canvas size. As the starfield image is 512x512, we have changed the width and height parameters in the game configuration, from the previous 700x700. The alternative would be to change the size of the tilesprite to 700x700, which would also be easy using something like MS 3D Paint.
Then the following code can be called from the update function to "scroll" the background.
stars.tilePositionY -= 1;
And that's it! Really simple!
Using Blitter object
The various bullet firing Phaser.Discourse examples that we have judiciously "leveraged" achieve the same effect using something called a Blitter object.
After loading the image in preload, just like with the tilesprite, the blitter object is created within the create function as follows.
stars = this.add.blitter(0, 0, "starfield");
stars.create(0, 0);
stars.create(0, -512);
Then the entire blitter object is "scrolled" with the following lines of code within the update function.
stars.y += 1;
stars.y %= 512;
Unlike the tilesprite object, a blitter object has no automatic repeat or wrap, hence the code is a little bit more complicated. However, drawing a blitter object is apparently faster than drawing tilesprites.
For my simple danmaku creations to date, this speed difference is not important, but it is useful to know.
As usual, the entire CodePen Pen is available below, for your perusal.
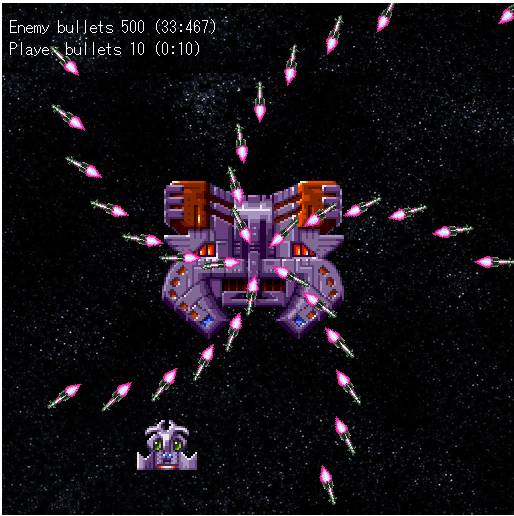
Comentarios