A relatively simple additional feature to the bullet class this time. We will give each bullet a "lifespan" after which it will disappear on its own, even if it is still within the play area.
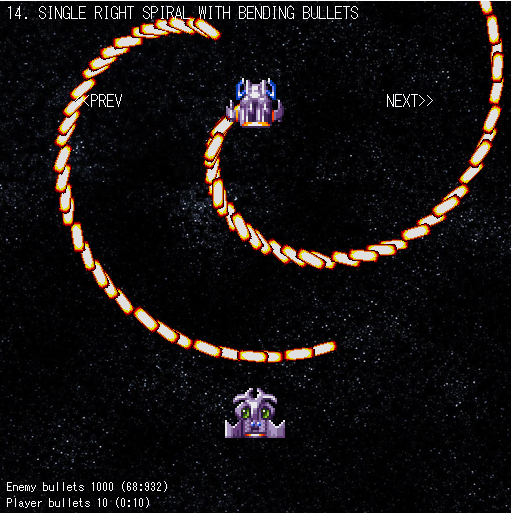
We will set up a number of new properties for the bullet object:
bulletLife: this will be the "life" of a bullet in milli-seconds. By default, it will be -1, which is meant to mean there is no finite life to the bullet.
timeSinceFired: exactly what it means. this will be time since the bullet was fired. This will be set to zero when the bullet object is activated.
Monitor the "life" of the bullet within preUpdate
We will utilise the preUpdate function once again. Within the preUpdate function, we will increment the timeSinceFired property by the time that has elapsed since last time the preUpadte function was called. Then we will compare that with bulletLife variable. If sufficient time has passed, then we will call the disableBody function.
I have at the same time turned the bunch of if statements that checked for the bulletType (between NORMAL and BEND, to switch/case statements to make it a little easier to read and maintain. But that's pretty much all the major changes.
preUpdate(time, delta) {
switch (this.bulletLife) {
case -1:
break;
default:
this.timeSinceFired += delta;
console.log(this.timeSinceFired, this.bulletLife)
if (this.timeSinceFired > this.bulletLife) {
this.disableBody(true, true);
}
break;
} ;
if (this.outOfScreen(this)) {
this.disableBody(true, true);
}
switch (this.bulletType) {
case "NORMAL":
break;
case "BEND":
this.scene.physics.velocityFromAngle(
this.angle,
this.body.speed,
this.body.velocity
); // reset velocity as bullet curves
this.scene.physics.velocityFromAngle(
this.angle,
this.bulletAcceleration,
this.body.acceleration
);
break;
}
} // end of preUpdate
Example danmaku pattern
If you combine it with a single spiral danmaku with strongly bending bullets, you get some an almost hypnotic effect where bullet streams will move almost snake-like around the screen and then disappear after a while.
Here's an example config for such a danmaku pattern.
danmakuPattern.push({
name: "SINGLE RIGHT SPIRAL WITH BENDING BULLETS",
danmakuType: "SINGLE",
numberOfCannons: 1, // number of (pair in case of bi-directional danmaku) cannons
cannonAngle: 90, // direction in which the primary cannon faces.
cannonAngularVelocity: 60,
numberOfRounds: -1, // how many times to shoot the weapon in succession
// properties for individual bullets
bulletType: "BEND",
bulletSpeed: 200, // speed of bullets fired from cannon
bulletAcceleration: 0,
bulletAngularVelocity: -90,
timeBetweenShots: 50, // time between bullets in ms
bulletLife: 6000,
bulletTexture: "bullet6" // specify the bullet image to be used,
});
As usual, the entire CodePen is available for your perusal.
コメント