Projections or the Dot Product
In the previous post, I explained the extremely useful Vector2 class built into Phaser 3. In this post, I explore the Dot product. The dot product makes an appearance so frequently in physics engines that a good understanding is particularly useful.
Projection
For those who want to remind themselves of the maths behind the dot product, the internet provides far better explanation that I ever could, including the wki here.
However, in terms of an intuitive explanation - the dot product can be thought of as projecting a point (or a from the origin) onto a line.
The following diagram (repeated from the previous post) shows the blue vector being projected onto the red vector. The end point of the blue vector, projected onto the red vector is shown by the green dot.
If you refer back to the wiki, it will give the following geometric definition of the product product of two Euclidean vectors a and b:

This should support the understanding of the diagram below.
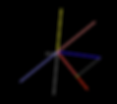
Mathematicians will cringe at the above explanation, but this intuitive understanding has gotten me pretty far in my implementation of physics engines!
The Phaser 3 implementation of the method is as follows. The dot product returns a scalar, ie it returns a number, not a vector
dot: function (src)
{
return this.x * src.x + this.y * src.y;
},
From a practical perspective, the dot product is useful in the following contexts:
The dot product of vector A and vector B, divided by the magnitude of vector B is the projection of A onto B.
The dot project of a vector with itself is the square of its magnitude.
If angle between the 2 vectors is less than 90 degrees, value of dot product is positive, if more than 90 degrees, negative
Dot product of 2 vectors that are perpendicular to each other is zero.
You will find the dot product very useful when determining how objects behave as they move around the screen, and if you look at the underlying code of relevant Phaser 3 methods, you will find dot product being used. It is useful to look at some of these.
Phaser.Geom.Point.Project(pointA, pointB [, out])
As the name suggests, this method literally is the dot product, except they are applied to Phaser.Geom.Point objects. It's a bit confusing to talk about dot product in terms of "points" but just think of point(x,y) = vector(x,y).
You will find that you can pass Phaser.Math.Vector2() to this method, and it will work perfectly find (although it will return a point object as opposed to a vector object).
var Project = function (pointA, pointB, out)
{
if (out === undefined) { out = new Point(); }
var dot = ((pointA.x * pointB.x) + (pointA.y * pointB.y));
var amt = dot / GetMagnitudeSq(pointB);
if (amt !== 0)
{
out.x = amt * pointB.x;
out.y = amt * pointB.y;
}
return out;
};
As an aside, you will note that the code calls GetMagnitudeSq, which is defined as follows:
var GetMagnitudeSq = function (point)
{
return (point.x * point.x) + (point.y * point.y);
};
The code above does away with the square rooting in the intermediary steps to work out the actual steps and only goes as far as to calculate the squared of the magnitude, but if you work through the maths, you will see that the end result is the same.
Useful dot product properties / identifies
I have poached from a selection of dot product properties which will become useful in understanding some of the logic as we develop our physics engine.
Commutative
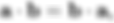
which follows from the definition (θ is the angle between a and b):

Distributive over vector addition

Orthogonal
Two non-zero vectors a and b are orthogonal if and only if a ⋅ b = 0.
Product rule
If a and b are (vector-valued) differentiable functions, then the derivative (denoted by a prime′) of a ⋅ b is given by the rule (a ⋅ b)′ = a′ ⋅ b + a ⋅ b′.
Useful References
https://youtu.be/-n_C7tD55_A (a practical look at the cross product in 2D)