Space Invaders in Phaser 3 (Part 6): Laying the Foundations
- cedarcantab
- Jan 11, 2022
- 2 min read
Updated: Jan 12, 2022
This post will be a very quick one describing the code to draw the "ground", before we move onto the much more challenging bits in the next post.
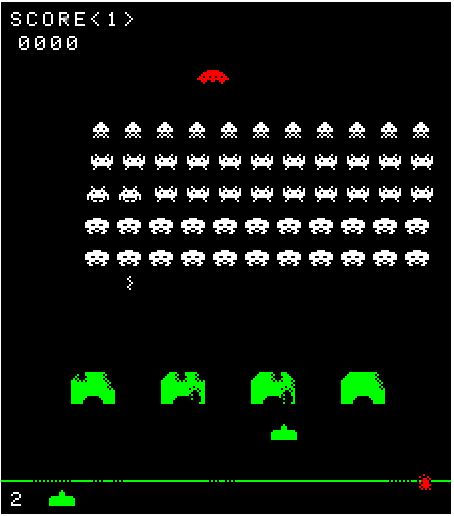
The Ground
In watching Youtube videos, one notices that there is a green line representing the ground. When an alien bomb reaches the ground, it explodes with the following image.

I cannot find the code which defines the duration of the explosion so I have assumed quite arbitrarily, 300 milli-seconds defined within class Bomb (to be covered in the following post).
As to the ground itself, the following code per CA offers some clues.
DrawBottomLine:
; Draw a 1px line across the player's stash at the bottom of the screen.
;
01CF: 3E 01 LD A,$01 ; Bit 1 set ... going to draw a 1-pixel stripe down left side
01D1: 06 E0 LD B,$E0 ; All the way down the screen
01D3: 21 02 24 LD HL,$2402 ; Screen coordinates (3rd byte from upper left)
01D6: C3 CC 14 JP $14CC ; Draw line down left side
As described in part 0, this code is setting up to draw a 1 pixel line at the 1st pixel of the 3rd byte which should be 17th pixel. In Javascript world this would translate to 239 (256 - 17).
After the bomb has exploded, the ground is seen "damaged". Frankly, I have no idea what the best way to achieve the same effect is in Phaser. In the end I simply drew a green line, using Phaser's built in Graphics gameobject methods. Similarly when the bomb reaches the bottom, I draw black graphic object points where the bomb touched the ground.
I created a "Ground" class object with its own methods for drawing the line and drawing 3 black dots along it.
class Ground {
constructor (scene) {
this.scene = scene;
}
static HEIGHT = 239;
layGround() {
this.scene.graphics.lineStyle(1,0x00ff00).lineBetween(0, Ground.HEIGHT, SCREEN_WIDTH, Ground.HEIGHT )
}
damage(x) {
this.scene.graphics.fillStyle(0x000000).fillPoint(x-2, Ground.HEIGHT);
this.scene.graphics.fillStyle(0x000000).fillPoint(x, Ground.HEIGHT);
this.scene.graphics.fillStyle(0x000000).fillPoint(x+2, Ground.HEIGHT);
}
}
Comments