Space Invaders in Phaser 3 (Part 10): SOUND
- cedarcantab
- Jan 16, 2022
- 3 min read
Sound can make a really big difference to the game experience. With Space Invaders, the thump thump sound as the the invaders move along the screen is very distinctive, particularly as the remaining number of aliens declines.
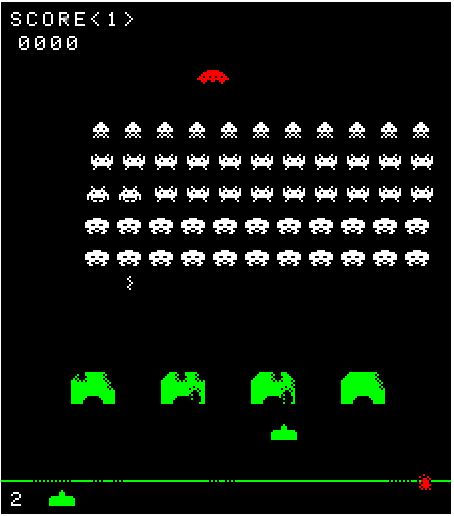
Whilst the Midway 8080 was revolutionary in having a CPU, it did not have a dedicated sound chip. Space Invaders used discrete circuits for its sound generation.
The Space Invaders sounds are all discrete analog circuitry. Opamps and decaying RC networks create an eerie realism that digital sound chips fall short of today. The individual sound circuits are triggered by bits in two output ports.
There appears to be a very deep community of people out there who "emulate" the sound generation in such hardware. However that is a very dark and long road. As such I have simply used sampled sound files to play at the appropriate time, by using Phaser's sound object play function for the majority of the effects. The background noise that the rack makes requires a bit of thought as it changes pace as the game progresses.
Fleet Tones
Computer Archeology has a comprehensive description of this works.
The speed of the fleet tones does NOT match the actual speed of the alien rack. The delay-between-tones is read from a table and depends on how many aliens are left in play.
; Alien delay lists. First list is the number of aliens. The second list is the corresponding delay.
; This delay is only for the rate of change in the fleet's sound.
; The check takes the first num-aliens-value that is lower or the same as the actual num-aliens on screen.
;
; The game starts with 55 aliens. The aliens are move/drawn one per interrupt which means it
; takes 55 interrupts. The first delay value is 52 ... which is almost in sync with the number
; of aliens. It is a tad faster and you can observe the sound and steps getting out of sync.
;
1A11: 32 2B 24 1C 16 11 0D 0A 08 07 06 05 04 03 02 01
1A21: 34 2E 27 22 1C 18 15 13 10 0E 0D 0C 0B 09 07 05
1A31: FF ; ** Needless terminator. The list value "1" catches everything.
The first value in the table is a delay of 52 interrupts between sounds when there are 50 or more aliens. When there are 55 aliens the step sounds are faster than the rack. When there are 50 aliens the step sounds are slower than the rack.
Javascript / Phaser 3 version
First I replicate the requisite tables as 2 different arrays, within the MainGame scene.
static FLEET_TONE_COUNT = [0x32, 0x2B, 0x24, 0x1C, 0x16, 0x11, 0x0D, 0x0A, 0x08, 0x07, 0x06, 0x05, 0x04, 0x03, 0x02, 0x01];
static FLEET_TONE_DELAY = [0x34, 0x2E, 0x27, 0x22, 0x1C, 0x18, 0x15, 0x13, 0x10, 0x0E, 0x0D, 0x0C, 0x0B, 0x09, 0x07, 0x05];
If you listen carefully you'll notice there are 4 different "tones" making up the background thump-thump. I create Phaser 3 sound objects for each and stick them in an array called this.fleeTones.
this.march1SFX = this.sound.add('march1SFX');
this.march2SFX = this.sound.add('march2SFX');
this.march3SFX = this.sound.add('march3SFX');
this.march4SFX = this.sound.add('march4SFX');
this.fleetTones = [this.march1SFX, this.march2SFX, this.march3SFX, this.march4SFX];
I declare 3 different variables to cycle through the different "tones" in accordance with the above table.
this.marchSoundDelay: the delay between each tone (as determined by the FLEET_TONE_DELAY array above)
this.marchSoundTimer: this is the counter used to track when to play the next tone
this.marchSoundCount: keep track of which of the 4 diffferent tones to play next
The code itself is fairly straightforward. This is called by the update function of the MainGame scene.
handleFleetTone() {
const index = MainGame.FLEET_TONE_COUNT.findIndex((x) => {return x<=this.rack.liveInvaders});
this.marchSoundDelay = MainGame.FLEET_TONE_DELAY[index];
this.marchSoundTimer ++;
if (this.marchSoundTimer >= this.marchSoundDelay) {
this.fleetTones[this.marchSoundCount].play();
this.marchSoundCount = (this.marchSoundCount < this.fleetTones.length-1) ? this.marchSoundCount+1 : 0;
this.marchSoundTimer = 0;
}
}
Here's the new code in CODEPEN.
Comments