MMOI of a Capsule
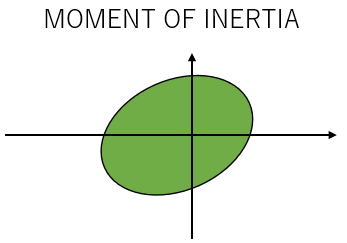
In this post we calculate the mmoi of a capsule.
To calculate the mmoi of a capsule, we consider it as the sum of a rectangle and two semi-circles. Then we can add the mmoi of each of the components and add them together.
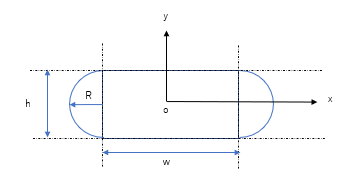
We already know the mmoi of a rectangle, around the z-axis through its centre of mass.
But what of a semi-circle? It is in fact, half the mmoi of a full circle - if this is not obvious, go back to how we derived the mmoi of a full circle in the previous circle, consider a semi-circle as consisting of very thin semi-circular rings. The "length" of a semi-circular ring is obviously half the length of a full "hoop". The rest of the derivation is the same - hence the mmoi is:

Then we can use the parallel axis theorem to add the mmoi of the elements together to arrive at the I of the total.

And that is quite enough algebra for one post.
Javascript Implementation
calculateMass(density = 0.005) {
// the mass of a capsule is the mass of the rectangular section plus the mass
// of two half circles (really just one circle)
const h = this.height;
const w = Phaser.Geom.Line.Length(this); // w is the distance between the centres of the end circles
const r2 = this.radius * this.radius;
// compute the rectangular area
let ra = this.height * w ; // area of the "rectangle";
let rm = ra * density; // mass of the rectangle area
let ca = Math.PI * r2 ; // area of the "circle area"
let cm = ca * density ; // mass of the circle area
this.area = ra + ca ; // total area of the capsule
this.mass = rm + cm; // total mass
this.inv_m = this.mass === 0 ? 0 : 1 / this.mass;
// inertia is the rectangular region plus the inertia of the 2 half circles at a distance from centre of mass
let d = w * 0.5; // distance of the centre of the "circle" from the centre of mass
// parallel axis theorem I2 = Ic + m * d^2
let cI = 0.5 * cm * r2 + cm * d * d;
let rI = rm * (this.height * this.height + w * w) / 12;
// add the rectangular inertia and cicle inertia
this.inertia = cI + rI;
this.inv_inertia = this.mass === 0 ? 0 : 1 / this.inertia;
}
Comments