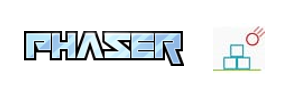
In the previous post, we got as far as detecting overlap between polygons using the separating axis theorem, and the clipping for contact points, then store them in a manifold in local coordinates. Before we take a look at how to transform these contact points to world coordinates, let us create a circle shape, and the methods to detect collision and create contact points.
BVef
class b2CircleShape extends b2Shape {
constructor(radius = 0.5) {
super()
this.m_type = b2Shape.e_circle;
this.m_radius = radius;
this.m_p = new Vec2(); // this is the center of mass local space
}
}
computeMass(density) {
this.m_mass.mass = density b2_pi this.m_radius this.m_radius;
this.m_mass.invMass = 1 / this.m_mass.mass;
this.m_mass.center = this.m_p;
// inertia about the local origin
this.m_mass.I = this.m_mass.mass (0.5 this.m_radius * this.m_radius + b2Dot(this.m_p, this.m_p));
this.m_mass.invI = 1 / this.m_mass.I;
}
computeAABB(aabb, transform) {
let p = Vec2.Add(transform.p, b2Mul(transform.q, this.m_p));//b2Vec2
aabb.lowerBound.set(p.x - this.m_radius, p.y - this.m_radius);
aabb.upperBound.set(p.x + this.m_radius, p.y + this.m_radius);
}
testPoint(transform, p) {
let center = Vec2.Add(transform.p, b2Mul(transform.q, this.m_p)); //b2Vec2
let d = Vec2.Subtract(p, center); //b2Vec2
return Vec2.Dot(d, d) <= this.m_radius this.m_radius;
}
Comments